Does React Context Replace React Redux?
17/06/2022
1.99k
Table of Contents
Table of contents
- What is Context?
- How and when to use a Context?
- When to use Redux?
- Does Context replace Redux?
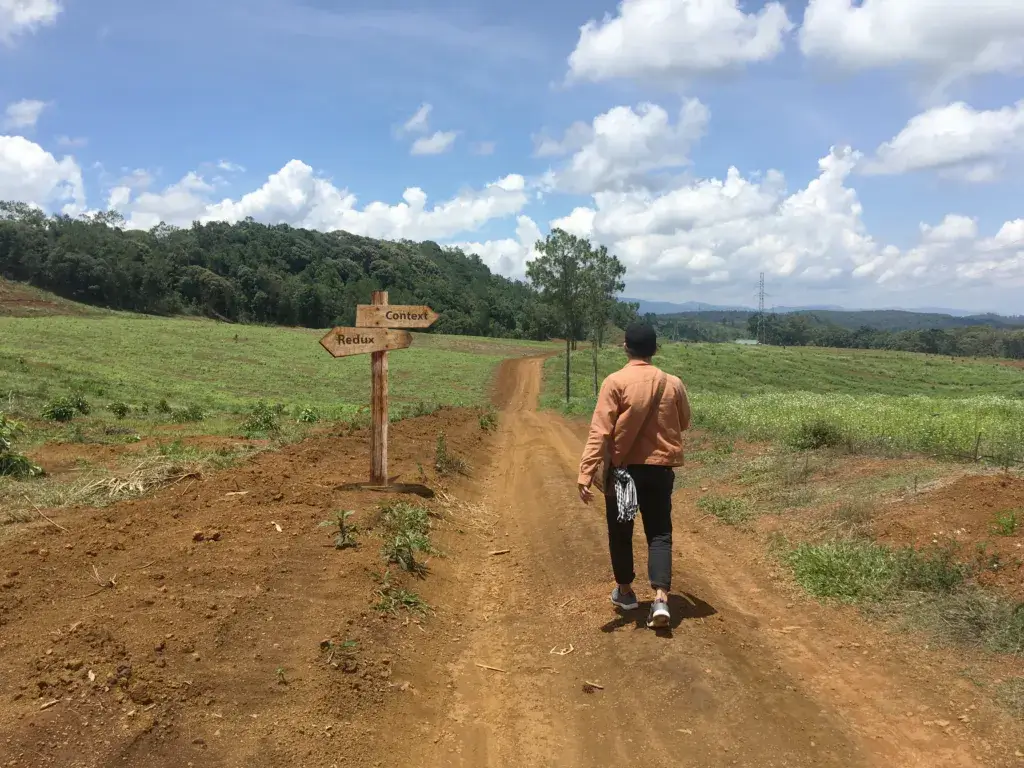
As you all know, nowadays, React has become one of the most famous JavaScript libraries in the world. It’s easy to learn and use. It comes with a good supply of documentation, tutorials, and training resources. Any developer who comes from a JavaScript background can easily understand and start creating web apps using React in a few days with the main concepts:
- JSX
- Rendering Elements
- Components and Props
- State and Lifecycle
- Handling Events
But to become an expert in React, it would take more time. You have to deep dive into advanced things:
- Higher-Order components
- Context
- Code-Splitting
- Avoiding unexpected rerender
- Especially, Effective state management
When it comes to state management in React applications, we instantly think about React Redux. This is a favorite and widespread tool across developer’s communities and applications. Recently, a lot of questions have come to us about using React Context as an alternative for React Redux. To answer those questions, have a brief of React Context first.
What is the context?
The context was originally introduced for passing props through the component tree, but since newer versions, React provided the powerful feature that allows updating context from a nested component. Meanwhile, Context can now be a state management tool. Let’s see how easy it works?
Context with Hooks
There are many ways to integrate Context, in this article, I just demo the simplest one
Create a context
import { createContext } from 'react'; export const ThemeContext = createContext(defaultTheme);
So simple, createContext
is the built-in function that allows you to create a context with default value.
Create a provider
A provider allow consuming components to subscribe to context changes
const { Provider } = ThemeContext; const ThemeProvider = ( { children } ) => { const theme = { ... }; return <Provider value={{ theme }}>{children}</Provider>; };
Create a consumer
The simplest way to subscribe to the context value is to use the useContext
hook.
export const ThemeInfo = () => { const { theme } = useContext(ThemeContext); return ( <div>backgroundColor: {theme?.background}</div> ); }
A consumer must be wrapped inside a Provider if not it would not be noticed and then re-render when context data changes.
<App> <ThemeProvider> <ThemeInfo></ThemeInfo> </ThemeProvider> </App>
Update the context from a consumer
It is sometimes necessary to update the context from consumers, context itself doesn’t provide any method to do that, but together with hooks, it can be easy:
- Use
useState
to manage context value setTime
is passed as a callback
const { Provider } = ThemeContext; const ThemeProvider = ( { children } ) => { const [theme, setTheme] = useState(defaultTheme); return <Provider value={{ theme, setTheme }}>{children}</Provider>; };
- From a consuming component, we can update the context value by executing the callback provided by context
export const ButtonChangeTheme = () => { const { setTheme } = useContext(ThemeContext); return ( <button onClick={() => setTheme(newTheme)}>Change</button> ); }
- Again, don’t forget to wrap
ButtonChangeTheme
consumer insideThemeProvider
<App> <ThemeProvider> <ButtonChangeTheme></ButtonChangeTheme> </ThemeProvider> </App>
When to use context?
As official guideline
Context is designed to share data that can be considered
global
for a tree of React components.
Below are common examples of data that Context can cover:
- Application configurations
- User settings
- Authenticated user
- A collection of services
How to use context in a better way?
- As mentioned before, when the context value changes, all consumers that use the context will be immediately re-rendered. So putting it all into one context is not a good decision. Instead, create multi-contexts for different kinds of data. EX: one context for
Application configurations
, one forAuthenticated user
and others. - Besides separating unrelated contexts, using each in its proper place in the component tree is equally significant.
- Integrate context adds complexity to our app by creating, providing, and consuming the context, so drilling props through 2-3 levels in a tree component is not a problem(Recommended to use instead of context)
- Restrictively apply context for high-frequency data updated, because context is not designed for that.
What about React Redux?
Unlike Context, React Redux is mainly designed for state management purposes, and covers it well.
However, take a look at some highlights from Redux team:
Not all apps need Redux. It’s important to understand the kind of application you’re building, the kinds of problems that you need to solve, and what tools can best solve the problems you’re facing.
You’ll know when you need Flux. If you aren’t sure if you need it, you don’t need it.
Don’t use Redux until you have problems with vanilla React.
Those recommendations clearly answer the question: When to use Redux?
Conclusion
So, Does React Context replace React Redux? The short answer is no, it doesn’t. React Redux and React Context can be used together instead of as alternatives. But how to use them together? It depends. If you understand the main concepts for each:
- React Context is designed for props drilling, it also has ways to update context data, but is recommended to deal with the simple follow of data changes.
- Redux is built for state management, especially for the frequently updated states used at many places in the application.
Based on that you can easily decide what should be implemented in your application.
In the end, try to use Context first, if something is not covered by Context, it’s time to use Redux.
Enjoy coding!
Reference
- Context official docs
- Redux FAQ: general
- If you are not interested in using Redux, you can check out React RetiX for a different approach to state management.
- I can not remember all the sources I have read, but it helps a lot in having comparison between Context and Redux. Thank you and sorry for not listing them all in this article!
Author: Vi Nguyen
Related Blog